Things you should learn before starting React
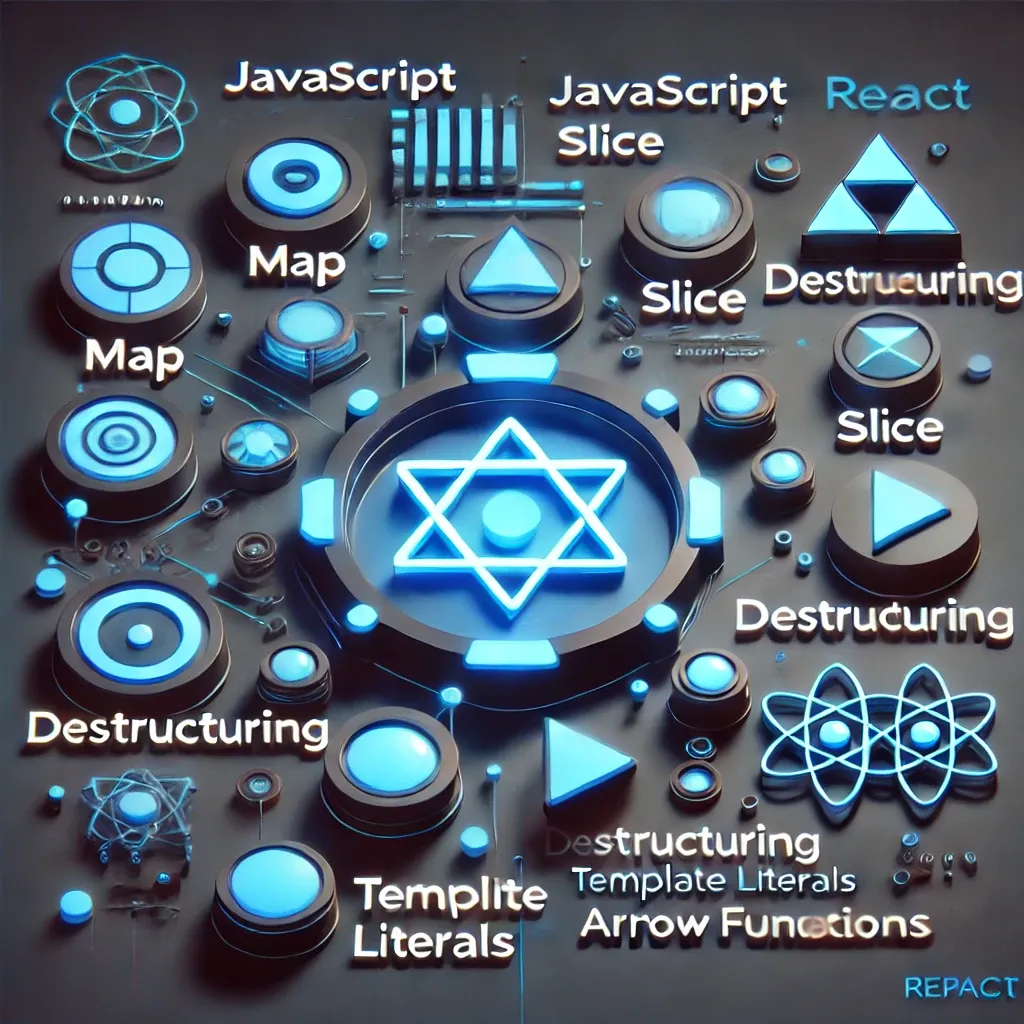
Arrow Functions
Arrow functions provide a concise way to write function expressions which are excellent for handling common tasks such as defining event handlers and creating function components
const sum = (a, b) => a + b;
console.log('The sum of 3 and 5 is ', sum(3, 5));
Map and Filter
Map creates a new array by transforming each element, while Filter creates a new array with elements that pass a test.
Map provides a concise and declarative to render html content and components in react from a list of data. Filter on the other hands removes unwanted data from a list
const numbers = [1, 2, 3, 4, 5, 6, 7]
const squares = numbers.map(el => el * el) // use of map to get squares of numbers
const even = numbers.filter(el => el % 2 === 0) // use of filter to get even numbers
Slice and Splice
Slice
Slice returns a shallow copy of a portion of an array without modifying the original array. It does not modify the contents of the original array. It returns a new array
const numbers = [1, 2, 3, 4, 5];
const slicedNumbers = numbers.slice(1, 4); // Extracts elements from index 1 to 3 (4 is not included)
console.log(slicedNumbers); // Output: [2, 3, 4]
console.log(numbers); // Output: [1, 2, 3, 4, 5] (Original array remains unchanged)
Splice
Splice modifies the original array by adding, removing or replacing elements.
const colors = ["red", "blue", "green", "yellow"];
const removedColors = colors.splice(1, 2); // Removes 2 elements starting from index 1
console.log(removedColors); // Output: ["blue", "green"]
console.log(colors); // Output: ["red", "yellow"] (Original array is modified)
const fruits = ["apple", "banana", "grape"];
fruits.splice(1, 0, "orange", "mango"); // Inserts at index 1 without deleting anything
console.log(fruits); // Output: ["apple", "orange", "mango", "banana", "grape"]
React's State Management Requires Immutability. In React, we should not modify state directly; instead, we create a new copy and update it. slice()
is useful for creating copies of state data, whereas splice()
modifies arrays in place (which is discouraged in React state updates).
Example: Bad Practice (Mutating State in React)
const [items, setItems] = useState(["apple", "banana", "cherry"]);
const removeItem = () => {
items.splice(1, 1); // Mutates the state directly
setItems(items); // React may not re-render properly
};
Example: Good Practice (Using slice)
const [items, setItems] = useState(["apple", "banana", "cherry"]);
const removeItem = () => {
setItems(items.slice(0, 1).concat(items.slice(2))); // Creates a new array
};
React's reconciliation process benefits from immutable data updates. Methods like slice()
help create new state objects without modifying the previous one, allowing React to detect changes efficiently.
Destructuring
Array Destructuring
Allows you extract elements from an array and assign to variables
// Basic Array Destructing
const numbers = [10, 20, 30];
// Extract values into separate variables
const [first, second, third] = numbers;
console.log(first); // Output: 10
console.log(second); // Output: 20
console.log(third); // Output: 30
// Skipping Elements
const colors = ["red", "blue", "green", "yellow"];
// Skip the second element
const [firstColor, , thirdColor] = colors;
console.log(firstColor); // Output: "red"
console.log(thirdColor); // Output: "green"
// Rest Operator
const fruits = ["apple", "banana", "cherry", "date"];
const [firstFruit, ...restFruits] = fruits;
console.log(firstFruit); // Output: "apple"
console.log(restFruits); // Output: ["banana", "cherry", "date"]
Object Destructuring
Used to extract values from objects and assign to variables
// Basic object destructuring
const person = { name: "Gillis", age: 29, country: "Cameroon" };
const { name, age, country } = person;
console.log(name); // Output: "Gillis"
console.log(age); // Output: 29
console.log(country); // Output: "Cameroon"
// Renaming variables
const user = { username: "gillis", email: "me@codewithgillis.com" };
const { username: userName, email: userEmail } = user;
console.log(userName); // Output: "gillis"
console.log(userEmail); // Output: "me@codewithgillis.com"
// Setting default values
const product = { title: "Laptop", price: 1000 };
const { title, price, stock = "Out of Stock" } = product;
console.log(stock); // Output: "Out of Stock"
Destructuring in Function Parameters
It can also be used directly in function parameters
function displayUser({ name, age }) {
console.log(`User: ${name}, Age: ${age}`);
}
const user = { name: "Alice", age: 30, location: "New York" };
displayUser(user); // Output: User: Alice, Age: 30
The above concepts are widely used in react when working with props and states in function components
function UserProfile({ name, age, location }) {
const [visits, setVisits] = useState(0); // Destructuring useState
return (
<div>
<h2>{name}</h2>
<p>Age: {age}</p>
<p>Location: {location}</p>
<p>Visits: {visits}</p>
</div>
);
}
// Usage
<UserProfile name="Gillis" age={29} location="Cameroon" />;
Template Literals
Template literals (also called template strings) are a feature in JavaScript that allow you to create strings more efficiently using backticks `
, instead of regular quotes ('
or "
). They also enable string interpolation, multiline strings, and embedded expressions.
const name = "John";
const greeting = `Hello, ${name}!`;
console.log(greeting); // Output: Hello, John!
In react, we use template literals extensively in several cases
- Easier JSX and String Handling
const name = "React";
return <h1>{`Welcome to ${name}!`}</h1>;
- Dynamic Class Names in JSX
const isActive = true;
return <div className={`button ${isActive ? "active" : "inactive"}`}>Click me</div>;
- Improved Readability in Styled Components
const StyledButton = styled.button`
background: ${props => (props.primary ? "blue" : "gray")};
color: white;
`;
- Simplifying API Calls and URLs
const userId = 123;
fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.then(data => console.log(data));
Conclusion
Learning these JavaScript features before React ensures:
✅ Better state & prop management (Destructuring)
✅ Simpler dynamic UI updates (map
, filter
, slice
, splice
)
✅ Efficient styling & string manipulation (Template Literals)
✅ More concise and readable functions (Arrow Functions)
✅ Clean & readable React code
By mastering these, transitioning to React will be much smoother and more intuitive! 🚀