Handle Your API Responses Like a Pro in Django
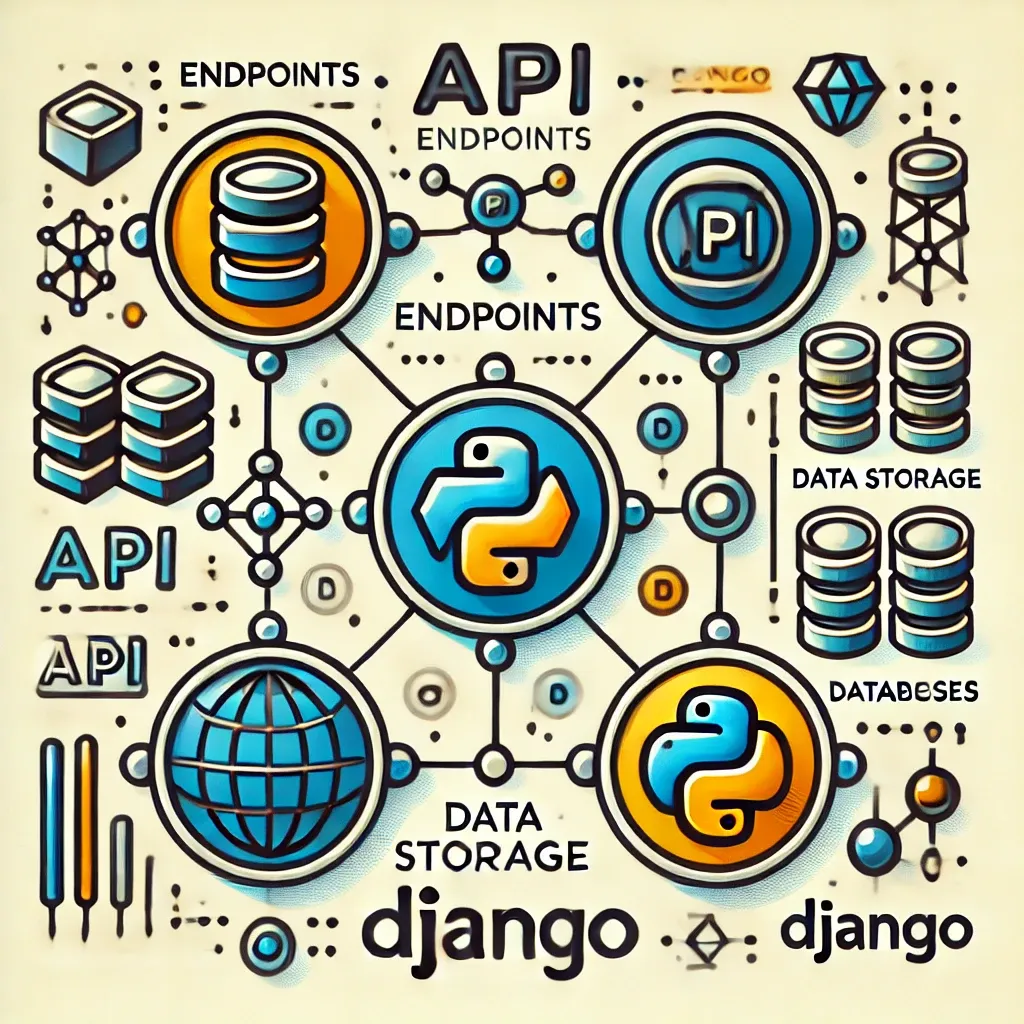
Introduction
When building APIs in Django, consistency in responses is key. A well-structured response format simplifies how the frontend handles API results, leading to a better developer experience and a smoother user experience.
A common approach is to structure API responses as:
{
"data": { /* Response Data as an object or list of objects and null if error */ },
"erc": 1, // 1 for success, other numbers for errors
"msg": "Associated message"
}
Why Is This Important?
✅ Predictability: The frontend can rely on a consistent format, reducing conditional checks.
✅ Standardized Error Handling: The UI can use the erc
value to determine the type of error without parsing complex error structures.
✅ Improved Debugging: Logs and API responses are uniform, making debugging easier.
✅ Scalability: If multiple teams work on the project, everyone follows a standard API response format.
Implementing a Middleware to Standardize API Responses
Instead of manually structuring responses in every view, we can use a Django middleware to automatically format all responses.
Step 1: Create the Middleware
class ApiResponseMiddleware:
def __init__(self, get_response=None):
self.get_response = get_response
def __call__(self, request):
response = self.get_response(request)
return response
def process_template_response(self, request, response):
if hasattr(response, "data") and not request.path.startswith("/api/schema"):
# add conditional checks above to ignore paths that have data in the response object but are not part of your api spec
if response.data and 'non_field_errors' in response.data:
response.data = {
'erc': response.data["non_field_errors"][0].code,
'msg': response.data["non_field_errors"][0]
}
elif response.data and response.status_code == 400:
print(response.data);
response.data = {
'erc': 0,
'msg': {key: ''.join([str(error_str) for error_str in value]) for key, value in response.data.items()}
}
elif response.data and response.status_code > 400:
print(response.data)
response.data = {
'erc': 0,
'msg': response.data.get('detail')
}
elif response.data and 'count' in response.data:
response.data = {
"erc": 1,
"msg": "success",
"total": response.data.get('count', 1),
"next": response.data.get('next'),
"data": response.data.get('results') if 'results' in response.data else response.data
}
else:
response.data = {
"erc": 1,
"msg": "success",
"data": response.data
}
return response
Step 2: Using an ErrorCode
Enum for Named Errors
To make error handling even more structured, we can use an ErrorCode
enum. This allows us to define named error codes with meaningful messages, making it easy to raise and handle errors concisely.
Defining the ErrorCode
Enum
from enum import Enum
from django.utils.translation import gettext_lazy as _
class ErrorCode(Enum):
INVALID_INPUT = (1001, _('Invalid input'))
AUTHENTICATION_FAILED = (1002, _('Authentication failed'))
PERMISSION_DENIED = (1003, _('Permission denied'))
RESOURCE_NOT_FOUND = (1004, _('Requested resource not found'))
SERVER_ERROR = (1005, _('Internal server error'))
def __init__(self, code: int, message: str):
self.code = code
self.message = message
How This Helps?
✅ Clear, Named Errors → Instead of returning raw numbers, we have readable, maintainable error codes.
✅ Standardized Error Responses → No need to manually define error messages in every view.
✅ Translation Support → gettext_lazy
enables internationalization for error messages.
Step 3: Using the ErrorCode
Enum in API Responses
With our middleware in place, Django views no longer need to manually format responses. The middleware will automatically wrap them in the {data, erc, msg}
structure.
The only responsibility of the views is to:
✅ Return data normally for successful requests.
✅ Raise structured errors using ErrorCode
for failures.
Example Usage in a View
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework.exceptions import ValidationError
from .error_codes import ErrorCode
class SampleView(APIView):
def get(self, request):
if "invalid_param" in request.query_params:
raise ValidationError(ErrorCode.INVALID_INPUT.message, ErrorCode.INVALID_INPUT.code) # Example of a validation error
return Response({"message": "Welcome!"}) # Middleware will wrap this
How the Middleware Handles Responses
For successful responses, the middleware automatically wraps the returned data:
View Returns:
{"message": "Welcome!"}
Middleware Converts to:
{
"data": { "message": "Welcome!" },
"erc": 1,
"msg": "Success"
}
For errors, the middleware extracts the ErrorCode
and formats the response:
View Raises:
raise ValidationError(ErrorCode.INVALID_INPUT.message, ErrorCode.INVALID_INPUT.code)
Middleware Converts to:
{
"data": null,
"erc": 1001,
"msg": "Invalid input"
}
Step 4: Activating the Middleware
Once the middleware is ready, add it to Django’s MIDDLEWARE
list in settings.py
:
MIDDLEWARE = [
...
'path.to.your.ApiResponseMiddleware', # Add this line
...
]
Final Thoughts
By implementing structured API responses and an ErrorCode
enum, you ensure:
✅ Consistent and readable responses for frontend developers
✅ Predictable error handling with predefined error codes
✅ Scalable and maintainable APIs
With this approach, you’ll handle API responses like a pro in Django! 🚀