Detect when your android application is idle
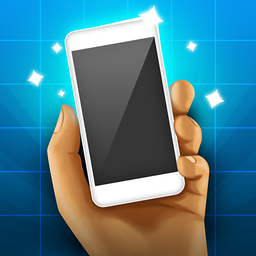
Sometime ago, while working on an android project, and I reached a point where I had to detect when my application is idle and perform a certain action. By idle here I mean the user has opened the app but is not interacting with.
What are we going to build?
Let’s assume a bank wanted to give it’s customers the ability to increase their account balances just by clicking a button 😃 (that might never happen, yes I know) and we need to build such an app for them. The user’s balance will reset to 0 if he delays for a period of time without interacting with the app. Simple app right? But remember our goal is to detect when the user has not been interacting with our app for sometime and not to build some fancy application. After this, you will be able to detect idle state of your application. Now enough of the talking. Let’s dive into some concepts and code.
We’ll start by creating a new android project on android studio. Feel free to call it whatever you want. I’ll provide a link to the repo at the end. Next we’ll create an empty activity and call it MainActivity. We’ll then create java class and call it BaseActivity. BaseActivity will be the parent class of all activities. The reason why I did this is because we’ll not want to write code in all our activities where we need to detect idle state of the application.
Let’s add some code to the BaseActivity class.
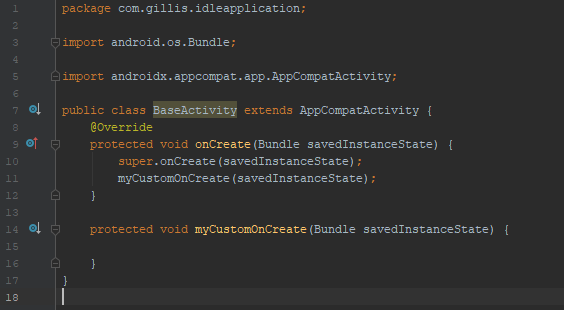
As you can see I created a method myCustomOnCreate that will be overidden by all child activities inorder to render the layout.
I already created the layout that allows the user add up what he has in account by simply tapping on a button. I have also implemented the java functionality. Remember that’s not purpose of this article so we’ll focus more performing an action when our app is idle. This is a look of our simple app.
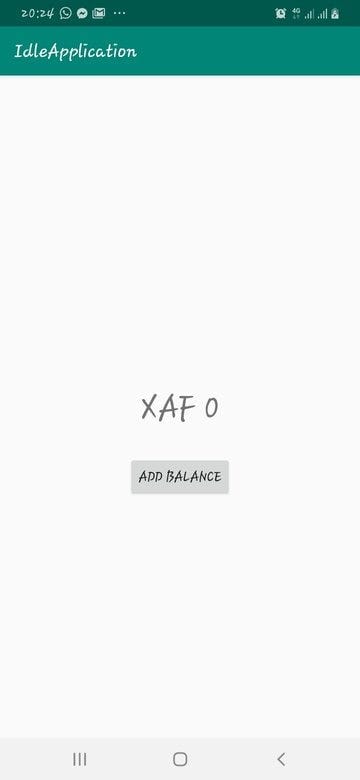
We will be using the android Handler class and Runnable interface. Inside the BaseActivity class, let’s create a handler object and pass a runnable object with a timeout to the handlers postDelayed. This will call the run method implementation inside our runnable implementation after timeout has occured. Let’s create a method and call it reactToIdleState. This method will be called inside the run method of the runnable implementation. We will also need to override this method inside all our Activities that extend BaseActivity. That is the method that will be called when the app becomes idle
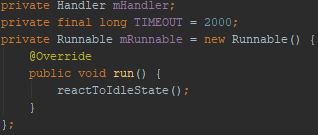
In the BaseActivity onCreate method, we will initialize our handler and call it’s postDelayed method passing into it the runnable object and the timeout as shown below. This simply means “Call the runnable’s run method after a timeout has reached”.
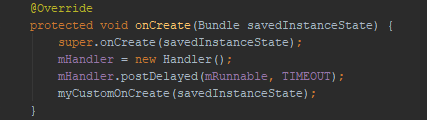
There are only two ways the user can interact with our app. Either by simple touching the screen or clicking the button to add their balance. So will make our BaseActivity class implement the View.OnClickListener interface and implement it’s onClick method. We will also the override the Activity onTouchEvent. In these two methods we will remove the callback from the handler by calling the removeCallbacks method and passing to it our runnable object, then call the postDelayed method again on the handler object. I have created a method called resetHandler to the job. We will call this method in our onClick and OnTouchEvent methods so every time user touches his screen or clicks the button we re-initialize the post delay.
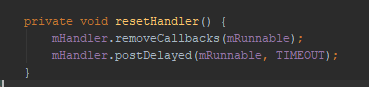
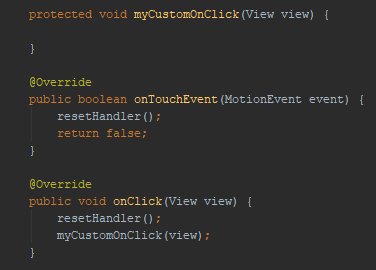
If you notice, in the onClick method I called a myCustomOnClick method that will be overridden inside all activities that extend BaseActivity and needs to respond to click events. That’s it for the BaseActivity. Now any of our activities can extend our BaseActivity and override the appropriate methods to perform actions on app idleness.
Our MainActivty will do the following
- Declare member variables and initialise them in the myCustomOnCreate method.
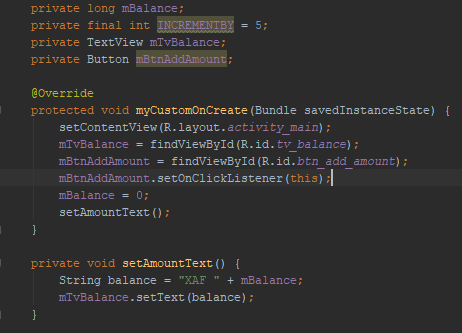
- React to the click events by overriding the BaseActivity myCustomOnClick method.
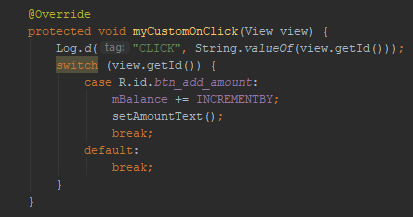
- Override the BaseActivity reactToIdleState method and perform desired action when the app is idle.
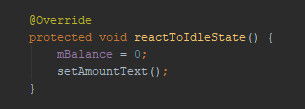
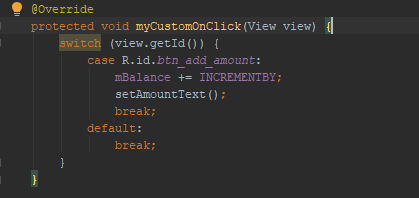
That’s it for our app. You can improve on the app functionality. But I would expect you to apply the concept to your own application where you need to know when the user hasn’t been interacting with the app for a while. Any idea about some other approach or questions will be highly welcome in the comment section. I’ll also be pleased if there could be corrections or something to add in the comment section. Here is the link to the complete project https://github.com/sonegillis/idle-state-detect-app. You can clone the project and run on your android device. To know more about Handlers and Runnable you can check out the following links to the google android developer site.
- https://developer.android.com/reference/android/os/Handler
- https://developer.android.com/reference/java/lang/Runnable